2 - Creating a Basic Star Chart
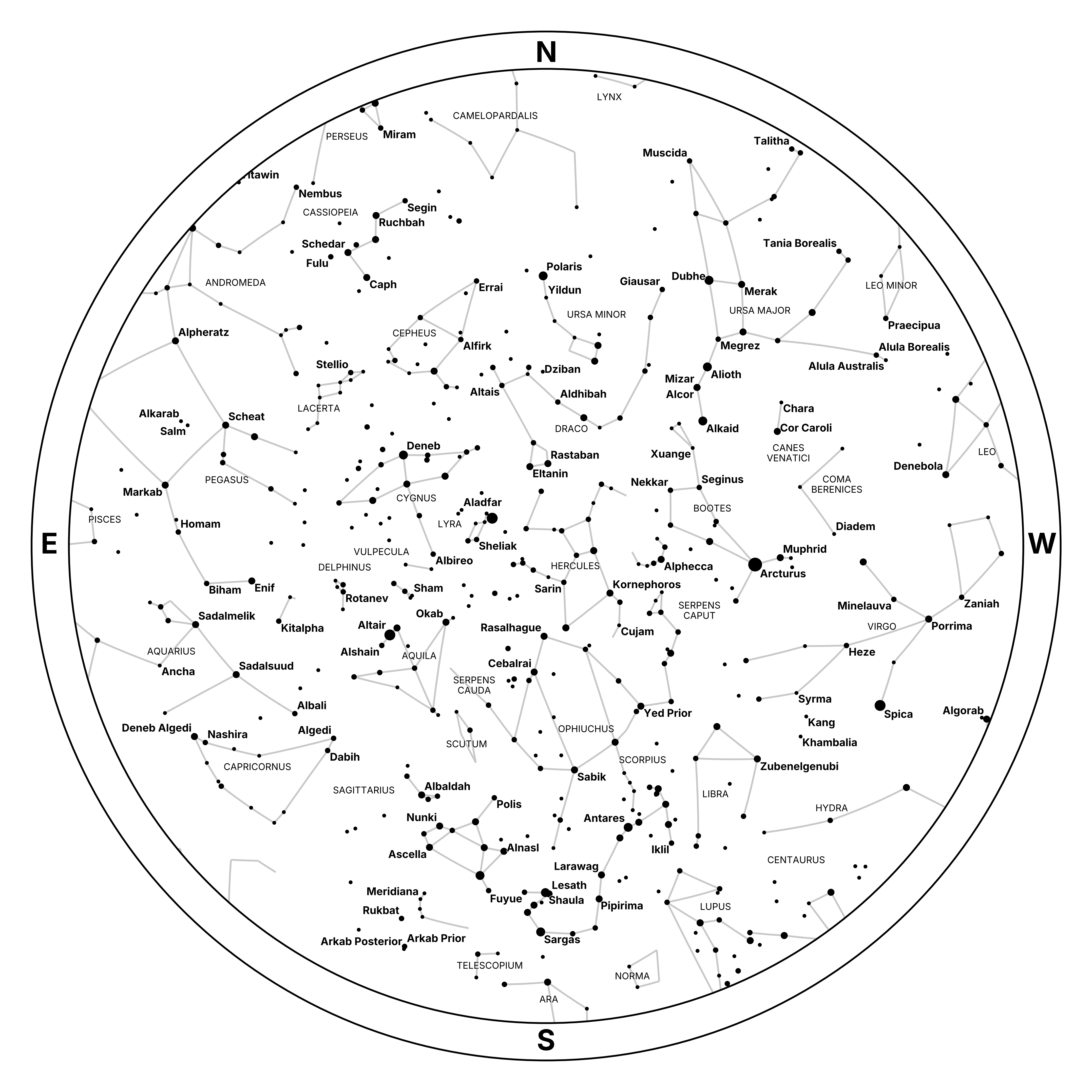
Let's begin our starplotting journey by creating a basic star chart that shows the entire sky at a specific time and place, like the chart above. Starplot calls these "Zenith" projections because the zenith is in the center of the chart.
Here's a look at the code to create the zenith chart above, which shows the sky as seen from Palomar Mountain in California on July 13, 2023 at 10pm PT:
In the first few lines, we just import all the stuff we need. pytz
is a required dependency of Starplot, so it should've been installed when you installed Starplot.
Next, we create a datetime
for our plot, including the timezone. All datetime
values in Starplot need to be timezone-aware. An exception will be raised if you try to pass a naive datetime.
On line 8, we create a MapPlot
instance that specifies a few properties for the map:
projection
: Method to use for transforming the curved shape of the three-dimensional sky to a flat two-dimensional map. In this example, we use aZENITH
projection, but Starplot supports many types of projections.lat
/lon
: Observing locationdt
: Observing date/timeresolution
: Resolution (in pixels) of the widest dimension of the plot
When you create a plot instance, initially it'll be empty (i.e. it won't have any stars). In Starplot, you have to explicitly plot all objects. So, on line 15 we plot the constellations, which includes lines and labels. Then, we plot stars with a limiting magnitude of 4.6.
Finally, we export the plot to a PNG file.
In the next section, we'll learn how to add some styles and other objects to the plot...