3 - Adding More Details to the Star Chart
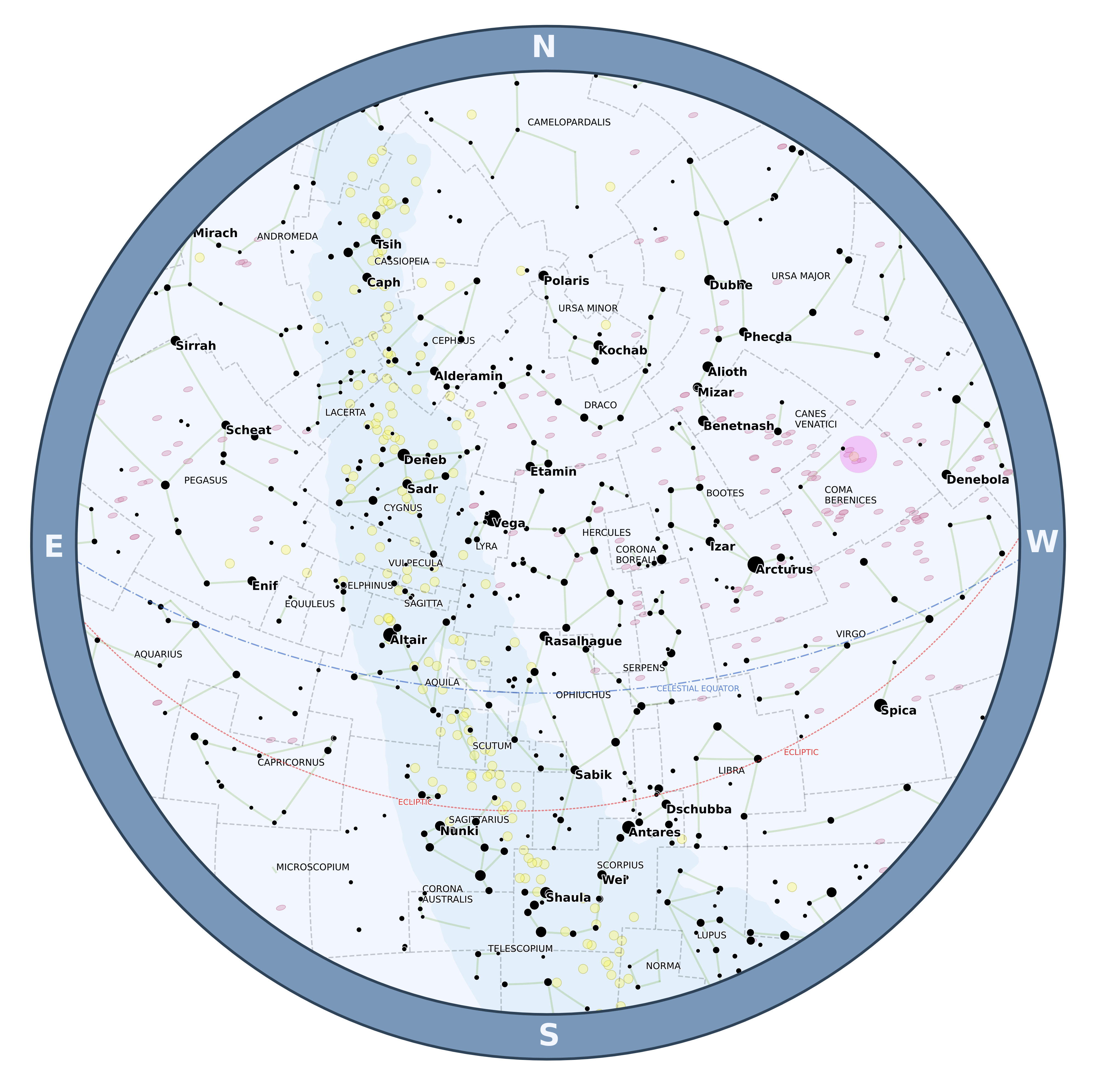
Building on the first example, let's add some color and more objects to the plot, to create the chart above. Here's the revised code with comments explaining the new lines:
Here are some more details about what we changed:
-
Customized the style by adding the
style
keyword argument when creating the plot instance. This is an instance of aPlotStyle
, and it represents ALL the styling properties to use for the plot (e.g. the colors, symbols, sizes, and more). In our revised example here, we create a defaultPlotStyle
and thenextend
it with theBLUE_MEDIUM
color scheme. Starplot has a very customizable styling framework that allows you to customize the appearance of just about anything you plot. -
Plotted deep sky objects (DSOs) with a limiting magnitude of 9. The
true_size=False
argument tells Starplot to plot each DSO's size based on what's specified in the style definition and NOT their true apparent size. For zenith plots like this one, plotting the true size can make many DSOs too small to see on the plot. We also passlabels=None
to hide all the labels for DSOs to avoid cluttering the plot. -
Plotted other lines and objects: constellation borders, ecliptic, celestial equator, and the Milky Way.
-
Added a marker for the Coma Star Cluster (aka Melotte 111), and customized its style. Starplot also has functions for plotting circles, rectangles, polygons, and more. See the reference for
MapPlot
for details.
In the next section, we'll learn how to create maps in other projections...